C++ Send Output To Dev Tty
- May 05, 2016 Feel free to use them! For example you could send a led state via address 1, an analog read via address 2, text chars for debug output via address 3 and other results like recieved ir signal via address 4. So much variation. You have up to 4byte (long) data to send, but it will automaticly only send the needed information (see how it works.
- Dec 11, 2015 Hmm, can you try connecting to the server with -tt option, e.g. Ssh -tt., or maybe even -ttt though I'm not sure if it makes any difference, but some people suggested using -ttt, so.
#include<stdio.h> |
#include<stdlib.h> |
#include<fcntl.h> |
#include<sys/stat.h> |
#include<sys/ioctl.h> |
#include<string.h> |
#include<unistd.h> |
voidprint_help(char *prog_name) { |
printf('Usage: %s [-n] DEVNAME COMMANDn', prog_name); |
printf('Usage: '-n' is an optional argument if you want to push a new line at the end of the textn'); |
printf('Usage: Will require 'sudo' to run if the executable is not setuid rootn'); |
exit(1); |
} |
intmain (int argc, char *argv[]) { |
char *cmd, *nl = 'n'; |
int i, fd; |
int devno, commandno, newline; |
int mem_len; |
devno = 1; commandno = 2; newline = 0; |
if (argc < 3) { |
print_help(argv[0]); |
} |
if (argc > 3 && argv[1][0] '-' && argv[1][1] 'n') { |
devno = 2; commandno = 3; newline=1; |
} elseif (argc > 3 && argv[1][0] '-' && argv[1][1] != 'n') { |
printf('Invalid Optionn'); |
print_help(argv[0]); |
} |
fd = open(argv[devno],O_RDWR); |
if(fd -1) { |
perror('open DEVICE'); |
exit(1); |
} |
mem_len = 0; |
for ( i = commandno; i < argc; i++ ) { |
mem_len += strlen(argv[i]) + 2; |
if ( i > commandno ) { |
cmd = (char *)realloc((void *)cmd, mem_len); |
} else { //i commandno |
cmd = (char *)malloc(mem_len); |
} |
strcat(cmd, argv[i]); |
strcat(cmd, ''); |
} |
if (newline 0) |
usleep(225000); |
for (i = 0; cmd[i]; i++) |
ioctl (fd, TIOCSTI, cmd+i); |
if (newline 1) |
ioctl (fd, TIOCSTI, nl); |
close(fd); |
free((void *)cmd); |
exit (0); |
} |
Copy the above code to some C file (For eg. ttyecho.c). Run the following command in the directory you have created the C file in to compile the code. |
make ttyecho |
Copy this file to the bin directory under your Home Directory. Create the directory if it doesn’t exist. Its a good practice to keep all custom binaries/executables in this bin directory. |
Start another terminal or switch to any other open terminal that you wish to control and execute the command tty. You can see a sample output below. |
@~$ tty |
/dev/pts/5 |
Now to execute a command on /dev/pts/5, run the following command in the controlling/original terminal. |
sudo ttyecho -n /dev/pts/5 ls |
You will see that the ls command is executed in /dev/pts/5. The -n option makes ttyecho send a newline after the command, so that the command gets executed and not just inserted. This utility can infact be used to send any data to other terminals For eg, you could open vim in `/dev/pts/5` and then run the following command in the controlling terminal to cause vim to exit in `/dev/pts/5`. |
sudo ttyecho -n /dev/pts/5 :q |
To avoid using sudo all the time, so that the command is easily scriptable, change the owners/permissions of this executable using the following commands. |
NB:Setting the setuid bit can become a security risk. |
sudo chown root:root ttyecho |
sudo chmod u+s ttyecho |
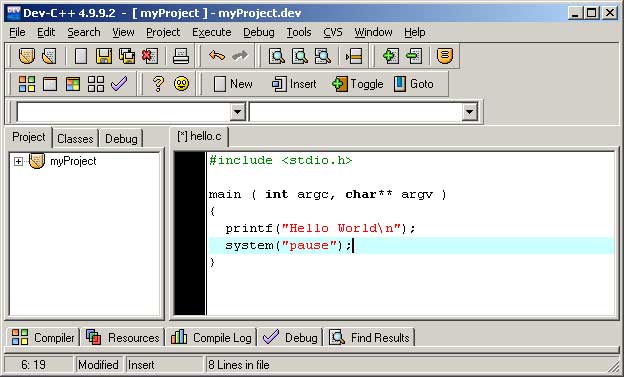
commented Oct 4, 2018
You just helped me so much! Cheers! |
Aug 24, 2011 C connecting to /dev/ttyUSB0 and r/w binary or hexadecimal data Hi, I'm trying to establish a connection from my Ubuntu 11.04 to a small project we've built together. Feb 10, 2009 Hi I am trying to (a) open a serial port, and then (b) write a stream of bytes to it and read any responses from the serial port. I have some code so far (will post below) but what I really want to know is, am I on the right track.
Syntax
Remarks
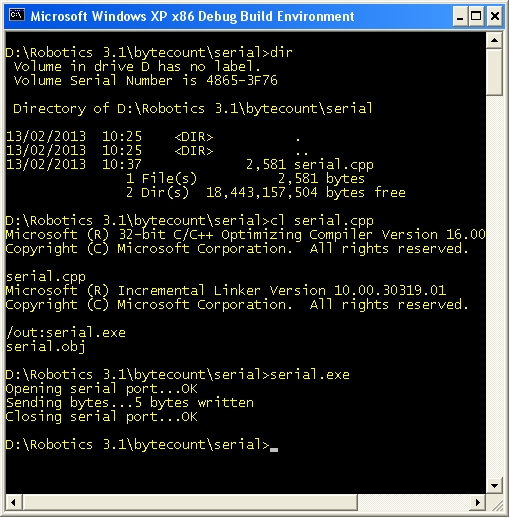
These are standard streams for input, output, and error output.
By default, standard input is read from the keyboard, while standard output and standard error are printed to the screen.
The following stream pointers are available to access the standard streams:
C++ Send Output To Dev Tty Number
Pointer | Stream |
---|---|
stdin | Standard input |
stdout | Standard output |
stderr | Standard error |
How to run step by step in dev c++. These pointers can be used as arguments to functions. Some functions, such as getchar and putchar, use stdin
and stdout
automatically.
These pointers are constants, and cannot be assigned new values. The freopen function can be used to redirect the streams to disk files or to other devices. The operating system allows you to redirect a program's standard input and output at the command level. Antares auto tune 7 crack full version.rar.
C Send Output To Dev Tty Code
See also
Dev Tty Keycaps
Stream I/O
Global Constants